PHP: Dependency inversion principle
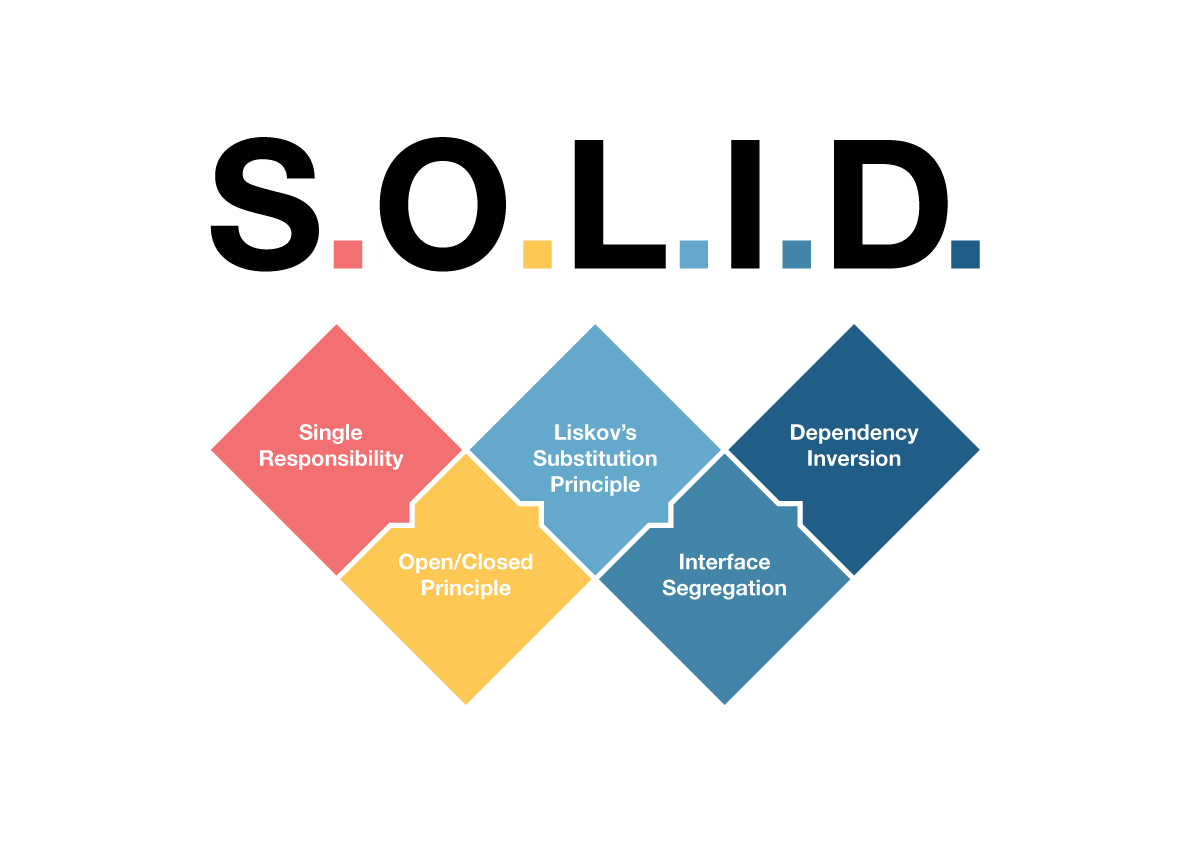
Definition
Dependency Inversion Principle (DIP), is one of the SOLID principles of object-oriented design. The Dependency Inversion Principle states that high-level modules (classes) should not depend on low-level modules; both should depend on abstractions. Additionally, abstractions should not depend on details; details should depend on abstractions. This principle encourages the use of interfaces or abstract classes to define contracts between different parts of your application.
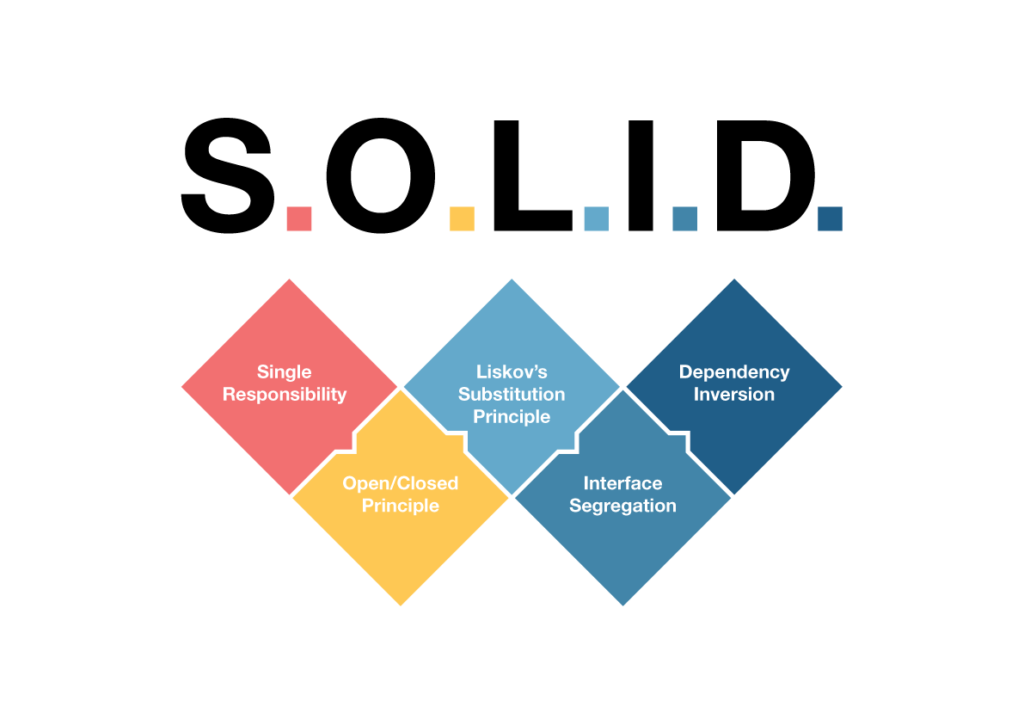
Here’s a simple PHP example to demonstrate the Dependency Inversion Principle:
Suppose we have a basic e-commerce system with two main classes: Order and EmailSender. The Order class is responsible for handling orders, and the EmailSender class is responsible for sending order confirmation emails.
Without following the Dependency Inversion Principle
class Order {
public function processOrder() {
// Process the order
$emailSender = new EmailSender();
$emailSender->sendEmail("Order processed successfully.");
}
}
class EmailSender {
public function sendEmail($message) {
// Code to send email
echo "Email sent: $message\n";
}
}
$order = new Order();
$order->processOrder();
In this example, the Order class directly creates an instance of EmailSender within its method, leading to tight coupling between the two classes.
Applying the Dependency Inversion Principle:
interface NotificationSender {
public function sendNotification($message);
}
class Order {
private $notificationSender;
public function __construct(NotificationSender $notificationSender) {
$this->notificationSender = $notificationSender;
}
public function processOrder() {
// Process the order
$this->notificationSender->sendNotification("Order processed successfully.");
}
}
class EmailSender implements NotificationSender {
public function sendNotification($message) {
// Code to send email
echo "Email sent: $message\n";
}
}
$order = new Order(new EmailSender());
$order->processOrder();
In this improved example, we’ve introduced an interface NotificationSender
that defines the contract for sending notifications. The EmailSender
class now implements this interface. The Order
class takes a NotificationSender
as a dependency through its constructor, adhering to the Dependency Inversion Principle. This makes it easier to change the way notifications are sent without modifying the Order
class.
Conclusion:
By adhering to the Dependency Inversion Principle, you create a more flexible and maintainable codebase where high-level modules depend on abstractions rather than concrete implementations, making it easier to replace or extend components without affecting other parts of the system.
Agile Agile Methodology AsyncStorage Dependency Inversion Principle Gutenberg Hooks Integration Testing JSON justify content center Justify text Migration Mindset Node Npm ObjectOriented design Performance Testing PHP React Js React Native React Native Buttons React Native Elements React Native Error React Native IOS React Navigation redux redux-persist redux-persist-react-native-async-storage Regression Testing Security Testing SOLID principles Unit Testing useDispatch User Acceptance Testing UAT WordPress WordPress Simplification WordPressVip WP Block Patters WP Gutenberg WP Gutenberg Patterns