Create & Extend Reusable Base Controller in CodeIgniter
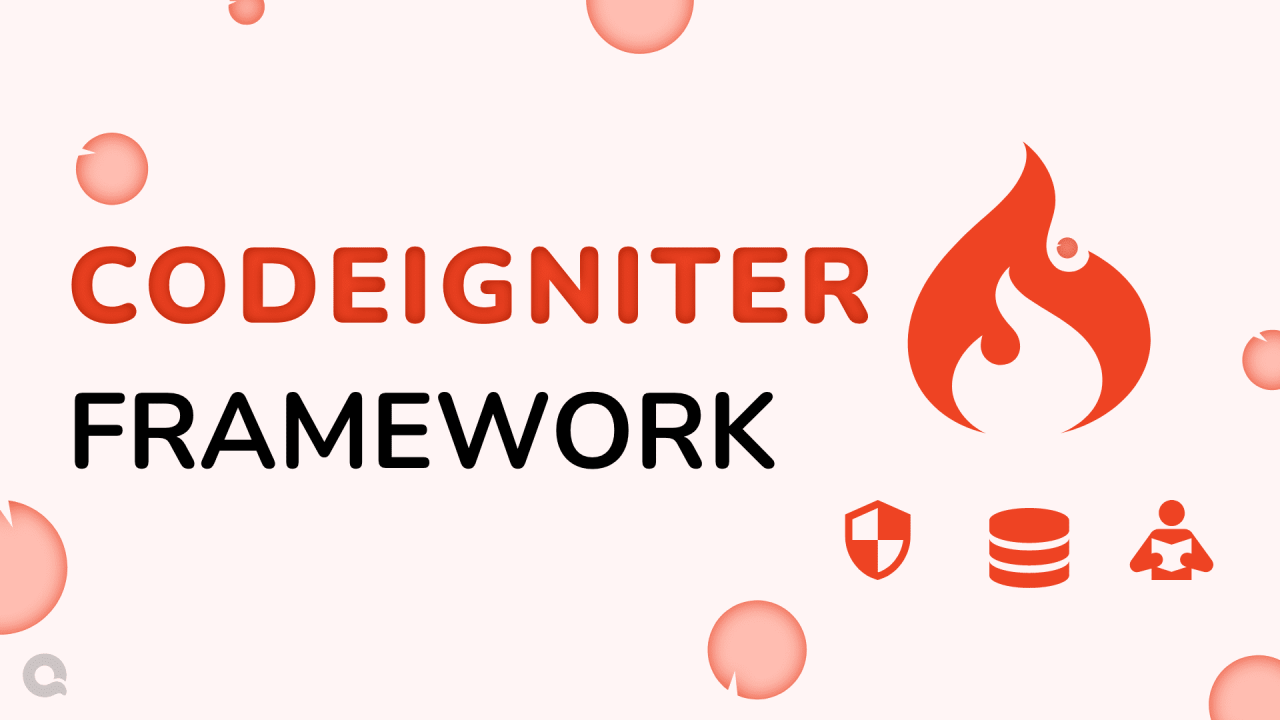
In CodeIgniter, you can create a base controller and then extend it to other controllers as a Reusable Base Controller. This approach is useful for sharing common functionality, like loading models, setting common properties, or including utility methods.
Here’s how you can do it:
Step 1: Create the Base Controller
- Create a new PHP file for your base controller in the
application/core
directory. Let’s name itMY_Controller.php
. - Define the base controller class. This class should extend
CI_Controller
.
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class MY_Controller extends CI_Controller {
public function __construct() {
parent::__construct();
// Load common models, libraries, helpers, etc.
$this->load->model('User_model');
// Additional common setup
}
// Add any common methods you want to use in all controllers
protected function render($view, $data = array()) {
$this->load->view('header', $data);
$this->load->view($view, $data);
$this->load->view('footer', $data);
}
}
Step 2: Extend the Base Controller in Your Controllers
- Modify your existing controllers to extend
MY_Controller
instead ofCI_Controller
.

User Controller Example
- Create or modify the controller in the
application/controllers
directory. Let’s useUser.php
as an example.
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class User extends MY_Controller {
public function __construct() {
parent::__construct();
// User-specific setup
}
public function show($id) {
$data['user'] = $this->User_model->get_user_by_id($id);
$this->render('user_view', $data);
}
public function index() {
$data['users'] = $this->User_model->get_all_users();
$this->render('users_view', $data);
}
}
Step 3: Create Views (If Necessary)
Ensure your view files are set up correctly in the application/views
directory.
Example View Files
user_view.php
<!DOCTYPE html>
<html>
<head>
<title>User Details</title>
</head>
<body>
<h1>User Details</h1>
<?php if ($user): ?>
<p>ID: <?php echo $user['id']; ?></p>
<p>Name: <?php echo $user['name']; ?></p>
<p>Email: <?php echo $user['email']; ?></p>
<?php else: ?>
<p>User not found.</p>
<?php endif; ?>
</body>
</html>
users_view.php
<!DOCTYPE html>
<html>
<head>
<title>All Users</title>
</head>
<body>
<h1>All Users</h1>
<?php if ($users): ?>
<ul>
<?php foreach ($users as $user): ?>
<li><?php echo $user['name']; ?> (<?php echo $user['email']; ?>)</li>
<?php endforeach; ?>
</ul>
<?php else: ?>
<p>No users found.</p>
<?php endif; ?>
</body>
</html>
header.php
<!DOCTYPE html>
<html>
<head>
<title>My Application</title>
</head>
<body>
footer.php
</body>
</html>
Summary
- Create a base controller (
MY_Controller.php
) inapplication/core
. - Extend the base controller in your specific controllers (
User.php
). - Use common methods defined in the base controller in your specific controllers.
By following these steps, you can create a base controller in CodeIgniter and extend it in other controllers, allowing for code reuse and better organization of common functionality.
Agile Agile Methodology AsyncStorage Dependency Inversion Principle Gutenberg Hooks Integration Testing JSON justify content center Justify text Migration Mindset Node Npm ObjectOriented design Performance Testing PHP React Js React Native React Native Buttons React Native Elements React Native Error React Native IOS React Navigation redux redux-persist redux-persist-react-native-async-storage Regression Testing Security Testing SOLID principles Unit Testing useDispatch User Acceptance Testing UAT WordPress WordPress Simplification WordPressVip WP Block Patters WP Gutenberg WP Gutenberg Patterns